What is it?
As we all know C# is a strongly typed language, and as we also know late binding 99.99% of the time is a really bad idea (performance and maintainability). To a certain extent the dynamic keyword is a step away from a strongly typed variable type. So what is the new dynamic keyword?
Lets have a look at an example:
namespace DynamicKeyword {
using System;
public class Program {
public static void Main(string[] args) {
Console.WriteLine("Test1");
dynamic variant = 123.44;
Console.WriteLine(variant);
Console.WriteLine(" of type " + variant.GetType().Name);
Console.WriteLine(" 9 + variant = " + (9 + variant));
variant = "hello world"; // This compiles ok too...
Console.WriteLine(variant);
Console.WriteLine(" of type " + variant.GetType().Name);
Console.WriteLine(" 9 + variant = " + (9 + variant));
Console.WriteLine(" " + variant.Length); // This compiles even though the Length property doesnt appear on the intellisense list.
}
}
}
The above code compiles and runs producing the following output.
Things to note:
- Assigning a double to a dynamic makes the variable type a double.
- Adding a number to it does proper number addition, not concatenation.
- Assigning a string to it compiles, and morphs the type to a string!
- Use of the + operation now performs string concatenation.
- We can access and call methods on the string type that were not there before when it was double.
Basically the dynamic keyword bypasses all of C#'s type checking.
You will still get exceptions at runtime to show inappropriate operations.
Consider this....
namespace DynamicKeyword {
using System;
public class Program {
public static void Main(string[] args) {
Console.WriteLine("Test1");
dynamic variant = 123.44;
Console.WriteLine(variant.Foo()); // Will compile but not work
}
}
}
Output at runtime:
Why is this potentially useful while making a mockery of strongly typed code?
- It could be useful for highly dynamic interfaces that are constantly changing.
- It could be useful for reinstantiation of json objects... See Pete's blog.
- Josh Smith suggested a using a proxy pattern to access any WPF UI element and using that element's dispatcher if necessary.
- Makes COM API's easier to consume. See this Msdn Article.
What is the difference between var and dynamic?
Easy. Var is strongly typed dynamic is not. Dynamic bypasses all of C#'s type checks at intelli-sense-time and at compile time.
Var simple is a macro for the compiler to insert the appropriate type name at compile time. The type is inferred from the right hand side of the assignment (=) operator.
Var makes code far easier to refactor and saves you typing. If you want to know the type simply hover the mouse over the var keyword it will tell you.
namespace DynamicKeyword {
using System;
public class Program {
public static void Main(string[] args) {
Console.WriteLine("Test1");
dynamic variant = "hello world";
Console.WriteLine(variant);
Console.WriteLine(" of type " + variant.GetType().Name);
variant = 123.44;
Console.WriteLine(variant);
Console.WriteLine(" of type " + variant.GetType().Name);
Console.WriteLine("Test2");
var notVariant = "Hello World Again!";
Console.WriteLine(notVariant);
notVariant = 123.45; // Does not compile
}
}
}
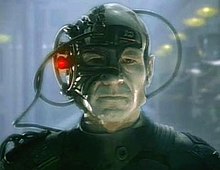
Resistance is futile.
Ben Rees - Jun 8, 2010 2:37 AM
Coincidently MSDN Mag has a good article on the Dynamic keyword this month also: http://msdn.microsoft.com/en-us/magazine/ff714583.aspx
Interesting idea to use it for COM interop, something I have not had to use a great deal, but does cover a gap comparing VB.Net to C# (with Vb's Option Strict feature).
Interesting idea to use it for COM interop, something I have not had to use a great deal, but does cover a gap comparing VB.Net to C# (with Vb's Option Strict feature).
Dynamic Programming - Understanding the Dynamic Keyword in C#4 (And the Expando object).
ReplyDeletehttp://msdn.microsoft.com/en-us/magazine/gg598922.aspx